The first project in 42 school was to create our own library, libft. We learnt the inner workings of standard C functions by coding over 40 functions from scratch. In this post, I’ll share what I’ve learnt and my approach for the project.
What are libraries?
When learning to code, one of your first programs would likely involve printing “hello world”. To achieve this, you’ll need to use a print function. But, how can we print something without having written the function?
This is where libraries come into play. Libraries contain prewritten code, including functions, to perform specific tasks such as copying strings and math calculations. With libraries, we can use code written by others before us instead of writing everything from scratch.
Hence, when printing text, we can use printf from the C library without having written the function.
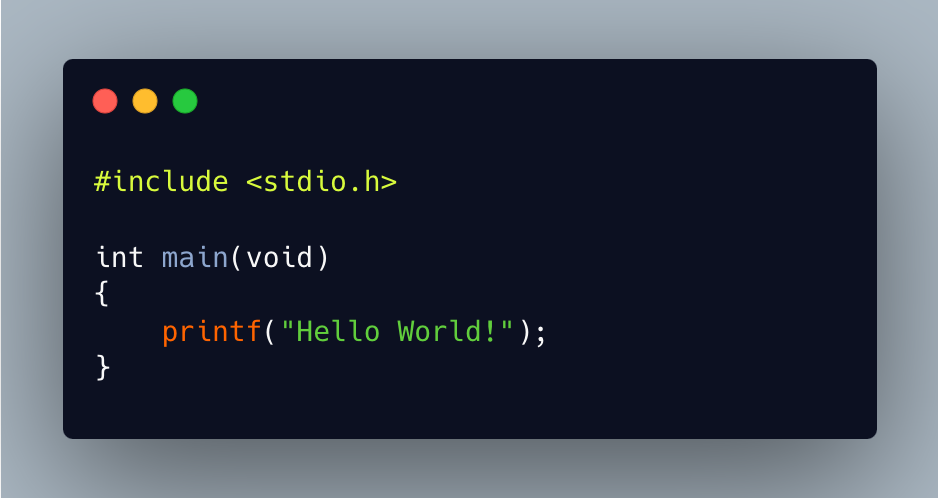
Learning by building
In the 42 curriculum, we’re not allowed to use most functions from the standard C library. Instead, we create our own library which will be used in later projects. The goal of libft is not only to replicate existing functions, but to understand how they work underneath the abstraction by building it ourselves.
Here are examples of the libft functions:
- ft_atoi converts a string into an int
- ft_memset fills x bytes of a string with an unsigned char value
- ft_strjoin concatenates the given strings and allocates memory for the new string
- ft_calloc allocates memory for count elements of size bytes and sets the memory to zero
- ft_lstadd_front adds a new node to the front of a singly linked list
My approach to creating libft
Starting the libft project felt like embarking on a challenging hike, with over 40 functions to complete, and validating the project felt like reaching the summit 🏔️
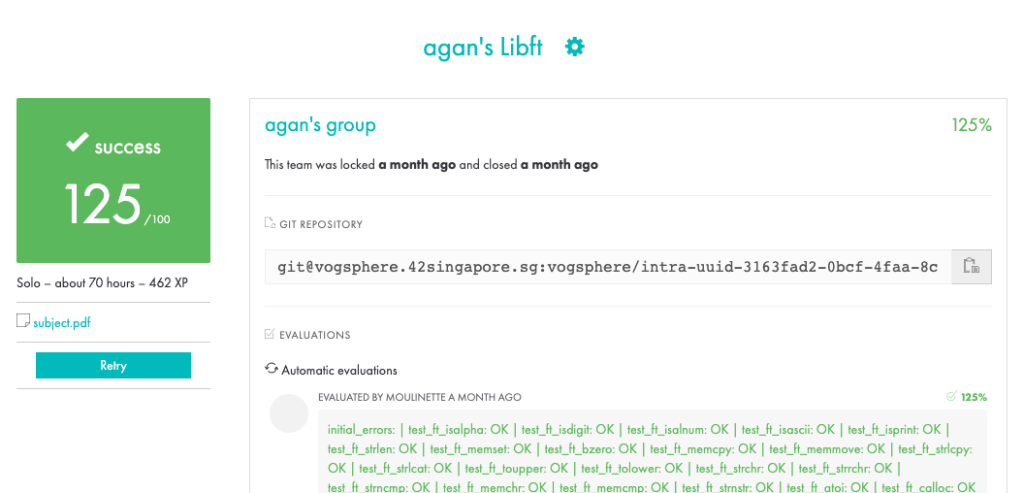
For the project, my approach centered on these elements: Navigating ambiguity, rigorous testing and leveraging peer-to-peer learning.
Navigating ambiguity
Diving into the sea of ambiguity, aka reading about the function from the man command was a challenge, as the description was often arcane. Here’s an example from the manual of strlcpy:
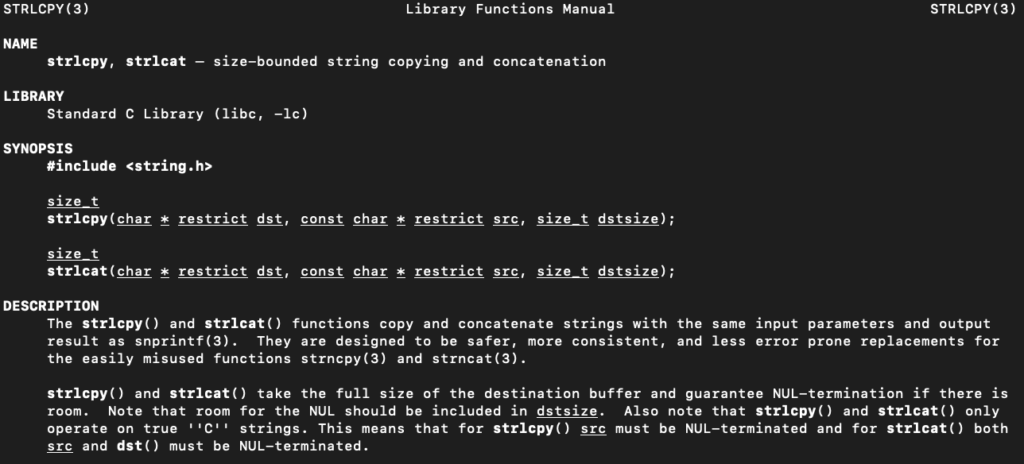
Personally, I found that my technical support experience was helpful as I was used to getting vague descriptions, breaking down problems and asking specific questions to get to the answers in my day-to-day work.
To start, we’d need to fully understand the function’s input and output, intended behavior and all possible return values. If the manual for the function is confusing, Google is your best friend to finding more beginner-friendly documentation. To build on this skill, I’d recommend not using ChatGPT as the first resource as:
- ChatGPT doesn’t always provide an accurate answer
- When you Google more often, you’ll learn to better filter information and know how to find the answers you need.
That isn’t to say that ChatGPT should not be used at all. It has helped me in aspects of debugging and improving efficiency, however when over-used it can hinder learning how to problem solve and source for answers.
Writing test cases
The most important part of this project for me was writing test cases. This project is evaluated by both peers and the automated grading system, Moulinette. Moulinette is a strict tester that would fail even a single typo – To ensure that we’re able to pass the rigorous testing, we’ll need to think of and test for edge cases, for instance when an empty string or invalid string is passed as an argument.
Additionally, writing rigorous test cases reinforces and affirms one’s understanding of the code. As an example, to test if someone understands addition, you might ask them the answer to 10 + 10 and expect 20. After all, if you do not understand addition, you wouldn’t know what question to ask or what answer to expect.
Peer-to-peer learning
Finally, learning from my peers has been an enriching process. When training as a technical support rep, my team lead advised that we ask for help if we’re stuck on the same ticket for 30 minutes. This is something that I think about often while coding – Knowing when to ask for help and what information to provide when doing so.
There were times I was stuck on the same error for almost an entire day. At the end of the day, I’d check with my peers and someone who have encountered a similar issue would be able to help resolve the error within minutes! The peer-to-peer learning culture not only makes learning interesting, but also less isolating.
Creating my own library was a thorough exercise; We learnt about the limitations and differences of the C library functions by meticulously planning, testing and debugging. Further, we learnt how to write reusable and readable code through constant feedback and exchange of ideas in peer evaluations.
If you have any feedback, feel free to drop them in the comments!
Leave a Reply